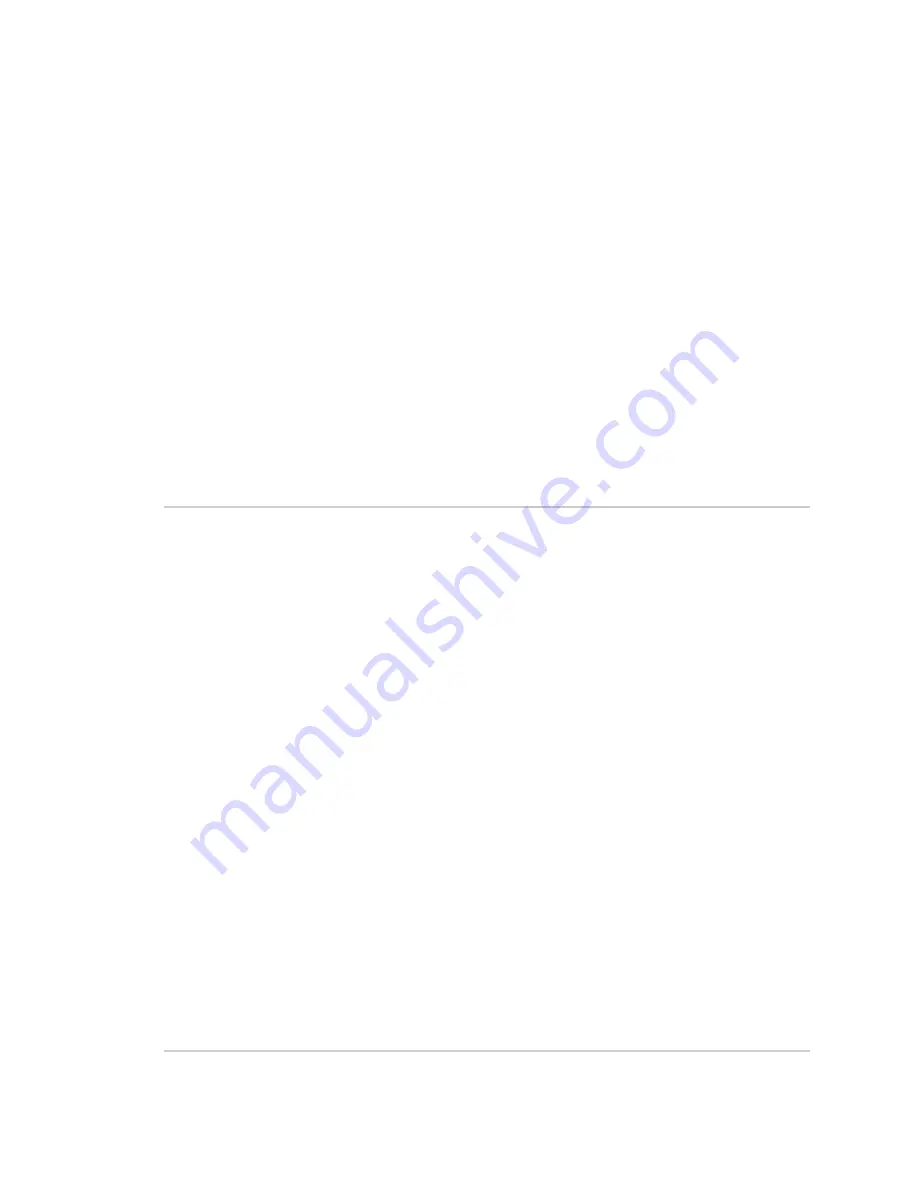
Get started with MicroPython
Example: code a request help button
Digi XBee3 Cellular LTE-M Global Smart Modem User Guide
53
Add the time the button was pressed
After you
add the ability to send an SMS
to the code, add functionality to insert the time at which the
button was pressed into the SMS that is sent. To accomplish this:
1. Create a UDP socket with the
socket()
method.
2. Save the IP address and port of the time server in the
addr
variable.
3. Connect to the time server with the
connect()
method.
4. Send
hello
to the server to prompt it to respond with the current date and time.
5. Receive and store the date/time response in the
buf
variable.
6. Send an SMS in the same manner as before using the
sms_send()
method, except that you add
the time into the SMS message, such that the message reads:
[Button pressed at: YYYY-MM-
DD HH:MM:SS]
To verify that your phone number is still in the memory, at the MicroPython
>>>
prompt, type
ph
and
press
Enter
.
If MicroPython responds with your number, copy the following code and enter it into MicroPython
using
and then run it. If it returns an error, enter your number again as shown in
text (SMS) when the button is pressed
. With your phone number in memory in the
ph
variable, copy
the code below and enter it into MicroPython using
and then run it.
from machine import Pin
import network
import usocket
import time
c = network.Cellular()
dio0 = Pin("D0", Pin.IN, Pin.PULL_UP)
while not c.isconnected():
# While no network connection.
print("Waiting for connection to cell network...")
time.sleep(5)
print("Connected.")
# Give feedback to inform user a button press is needed.
print("Waiting for SW2 press...")
while (1):
if (dio0.value() == 0):
# When button pressed, now the module will send "Button Press" AND
# the time at which it was pressed in an SMS message to the given
# target cell phone number.
socketObject = usocket.socket(usocket.AF_INET, usocket.SOCK_DGRAM)
# Connect the socket object to the web server specified in "address".
addr = ("52.43.121.77", 10002)
socketObject.connect(addr)
bytessent = socketObject.send("hello")
print("Sent %d bytes on socket" % bytessent)
buf = socketObject.recv(1024)
# Send message to the given number.
Handle error if it occurs.
try:
c.sms_send(ph, 'Button Pressed at: ' + str(buf))
print("Sent SMS successfully.")
except OSError:
print("ERROR- failed to send SMS.")
# Exit the WHILE loop.
break