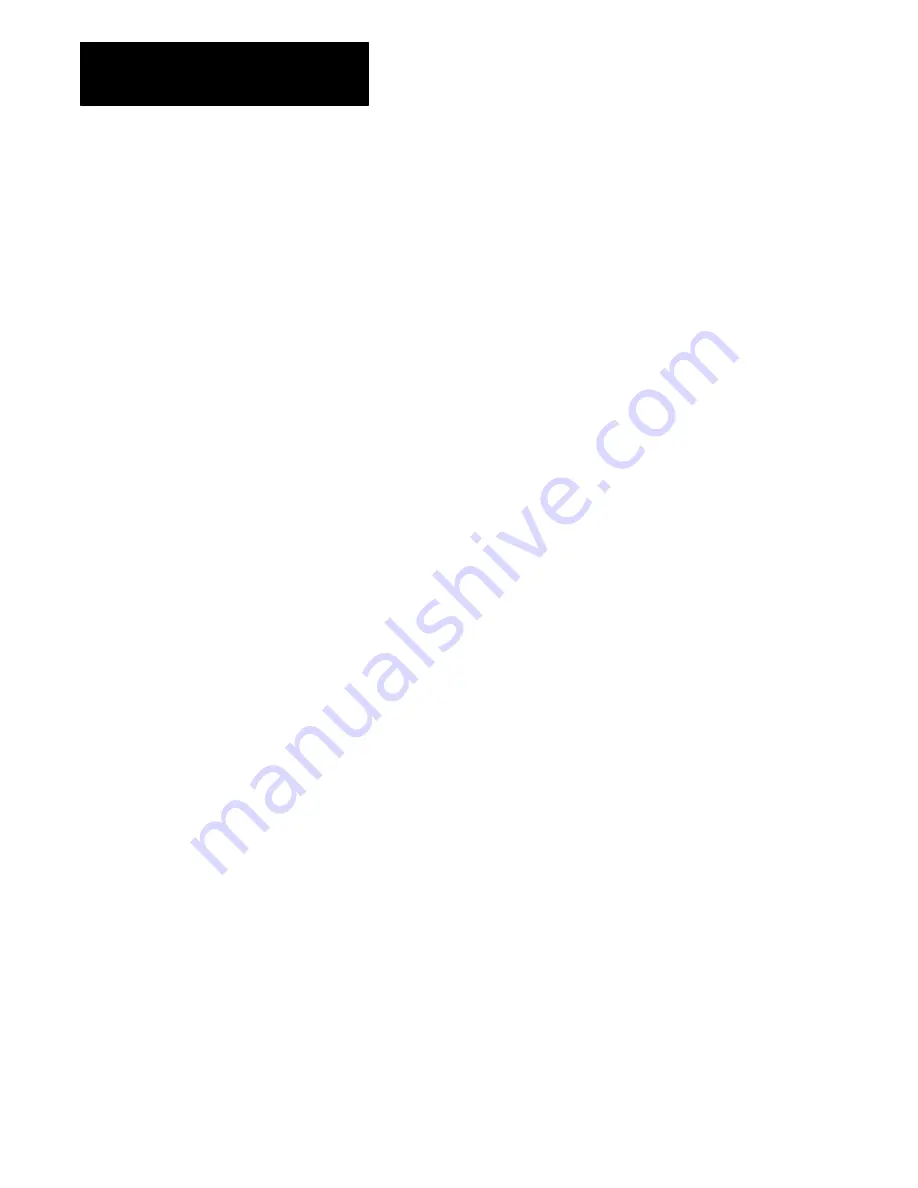
Programming Examples
Appendix B
B-32
load.c file
/*****************************************************************************
**
** FILE – load.c
**
** This file contains a routine for downloading the scanner binary into
** KTx memory.
**
*****************************************************************************/
#include<stdio.h>
#include<mem.h>
#include<dos.h>
#include <fcntl.h>
#include <io.h>
#include<process.h>
#include<string.h>
#include <conio.h>
#ifndef FALSE
#define FALSE 0
#define TRUE ~FALSE
#endif
/*****************************************************************************
GENERAL KTX DUAL PORT OFFSETS
*****************************************************************************/
#define KTX_RELEASE 0x802 /* Release the KTX (start it running) */
#define KTX_RESET 0x803 /* Reset the KTX (stop it) */
/*****************************************************************************
LOADPCL.BIN DUAL PORT OFFSETS
*****************************************************************************/
#define LOADPCL_DATA_WINDOW 0x80
#define LOADPCL_HANDSHAKE 0x75
#define LOADPCL_SIZE 0x72
#define LOADPCL_LENGTH 0x70
#define LOADPCL_STATUS 0x74
void usage(char *argv[]);
int main(int argc, char *argv[])
{
char far *base;
int address = 0,length,firstpass = 1;
int i,loadfile,ifile,file_into_dp = FALSE;
unsigned char loadfile_name[80] = {””};
/* Parse the command line for valid switches:
–fnnnnnnnn.eee ––> file name to download
–d ––> load file directly to dual port (diagnostics)
–axx ––> xx = upper byte of address of KTX (D3 e.g.)
*/
for (i = 1; i < argc; i++) {
if (argv[i][0] == ’–’)
switch(argv[i][1]) {
case ’f’:
case ’F’: /* Copy string into file name */
strcpy(loadfile_name, &argv[i][2]);
break;
case ’d’:
case ’D’: /* Set flag to copy directly to dual port */
file_into_dp = TRUE;
break;