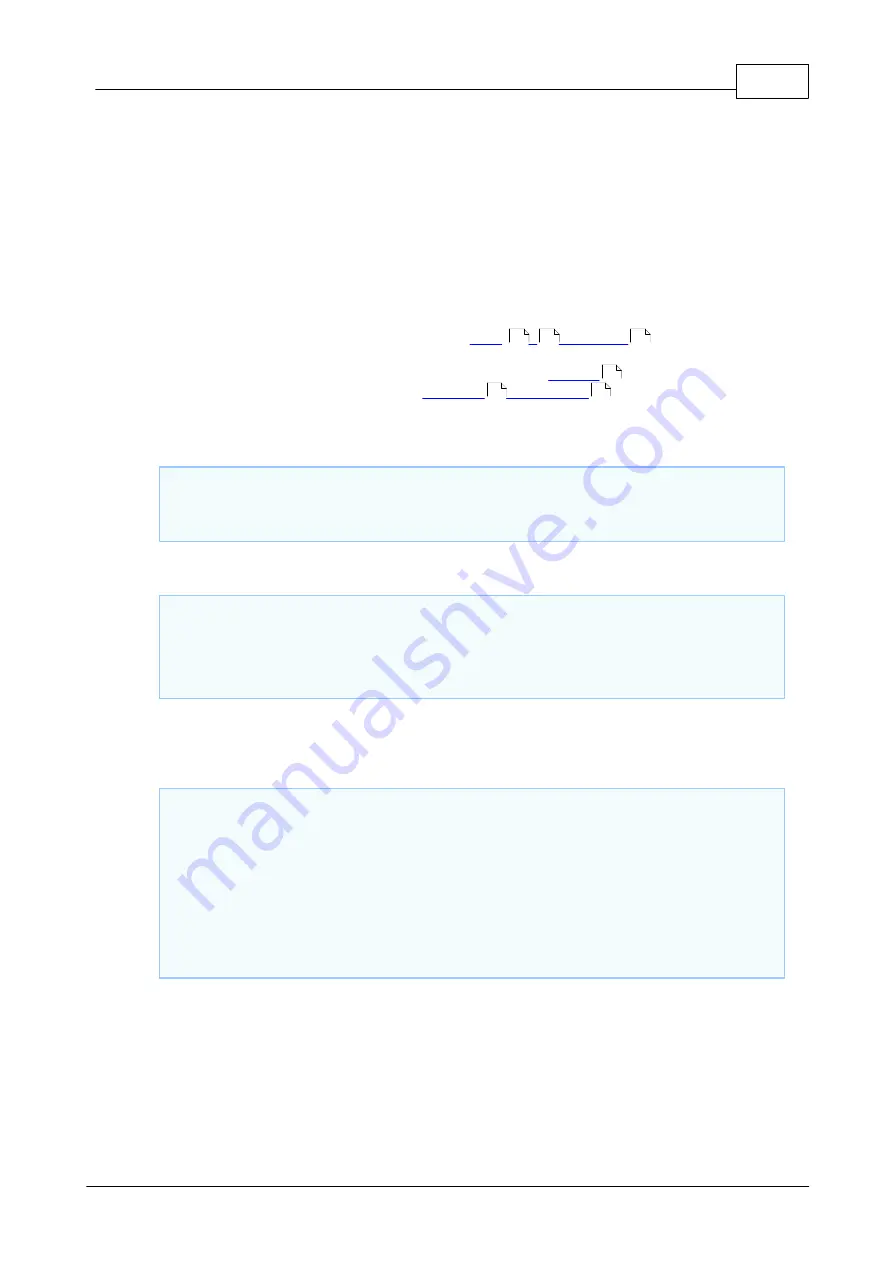
60
Programming with TIDE
©2000-2008 Tibbo Technology Inc.
functions from the HTML file. So, which way is better?
Generally, we recommend to use the second way. First of all, this style of
programming is cleaner- a mixture of BASIC code and static HTML text usually
looks messy. Second, the second method consumes less variable memory.
Although the HTML scope is similar to a local scope of a function or a sub, its
variables get exclusive memory allocation as if they were global. When you avoid
writing a lot of BASIC code in the HTML file itself you usually avoid having to
create a lot of variables in the HTML scope and this saves you memory!
4.2.4.9
Declaring Variables
Usually, a variable is first defined by the
and then used in
code; however, at times, a single global variable must be accessible from several
compilation units. In such cases, you must use the
modifier when
defining this variable, and use the
in each compilation
unit from which this variable needs to be accessed.
For example, let us say this is the file foo.tbs:
public
dim
i
as
integer
' the integer i is defined in this file, and made
into a public variable. It can now be used from other compilation units.
And this is the file bar.tbs:
i = i +
5
' this would cause a compiler error. What is i?
' the correct way:
declare
i
as
integer
' lets the compiler know that i is defined elsewhere.
i = i +
5
Also, if for some reason you would attempt to use a variable in a single compilation
unit before defining it using the dim statement, you will have to use a declare
statement before using it to let the compiler know that it exists. For example:
declare
i
as
integer
i =
7
' i has not been defined yet, but we let the compiler know that it
is defined elsewhere.
...
dim
i
as
integer
' we now define i. Note that here it doesn't have to be
public, because it is used in the same compilation unit.
' this example is rather pointless, but just illustrates this single
principle.
4.2.4.10
Constants
Constants are used to represent values which do not change throughout the
program; these values may be strings or numbers. They may either be stated
explicitly, or be derived as the result of an expression.
Some examples:
81
81
81
98
79
79