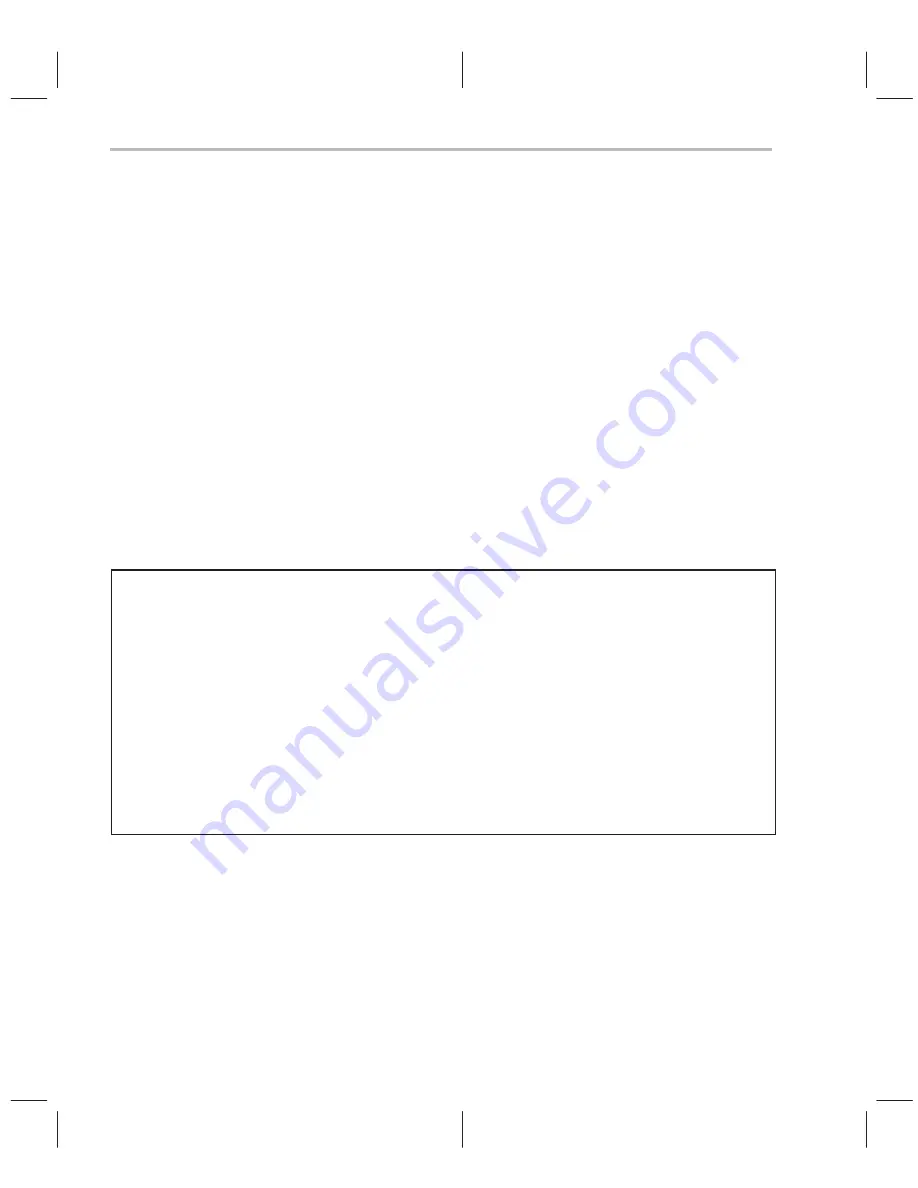
Lesson 1: Loop Carry Path From Memory Pointers
2-8
A If all of these pointers point to different locations in memory there is no de-
pendency. However, if they do, there could be a dependency.
Because all three pointers are passed into lesson_c, there is no way for the
compiler to be sure they don’t alias, or point to the same location as each other.
This is a memory alias disambiguation problem. In this situation, the compiler
must be conservative to guarantee correct execution. Unfortunately, the re-
quirement for the compiler to be conservative can have dire effects on the per-
formance of your code.
We know from looking at the main calling function in tutor_d.c that in fact, these
pointers all point to separate arrays in memory. However, from the compiler’s
local view of lesson_c, this information is not available.
Q How can you pass more information to the compiler to improve its perfor-
mance?
A The next example, lesson1_c provides the answer:
Open lesson1_c.c and lesson1_c.asm
Example 2–5. lesson1_c.c
void lesson1_c(short * restrict xptr, short * restrict yptr, short *zptr,
short *w_sum, int N)
{
int i, w_vec1, w_vec2;
short w1,w2;
w1 = zptr[0];
w2 = zptr[1];
for (i = 0; i < N; i++)
{
w_vec1 = xptr[i] * w1;
w_vec2 = yptr[i] * w2;
w_sum[i] = ( w_vec2) >> 15;
}
}
The only change made in lesson1_c is the addition of the restrict type qualifier
for xptr and yptr. Since we know that these are actually separate arrays in
memory from w_sum, in function lesson1_c, we can declare that nothing else
points to these objects. No other pointer in lesson1_c.c points to xptr and no
other pointer in lesson1_c.c points to yptr. See the
TMS320C6000 Optimizing
C/C++ Compiler User’s Guide for more information on the restrict type qualifi-
er. Because of this declaration, the compiler knows that there are no possible
dependency between xptr, yptr, and w_sum. Compiling this file creates feed-
back as shown in Example 2–6, lesson1_c.asm: