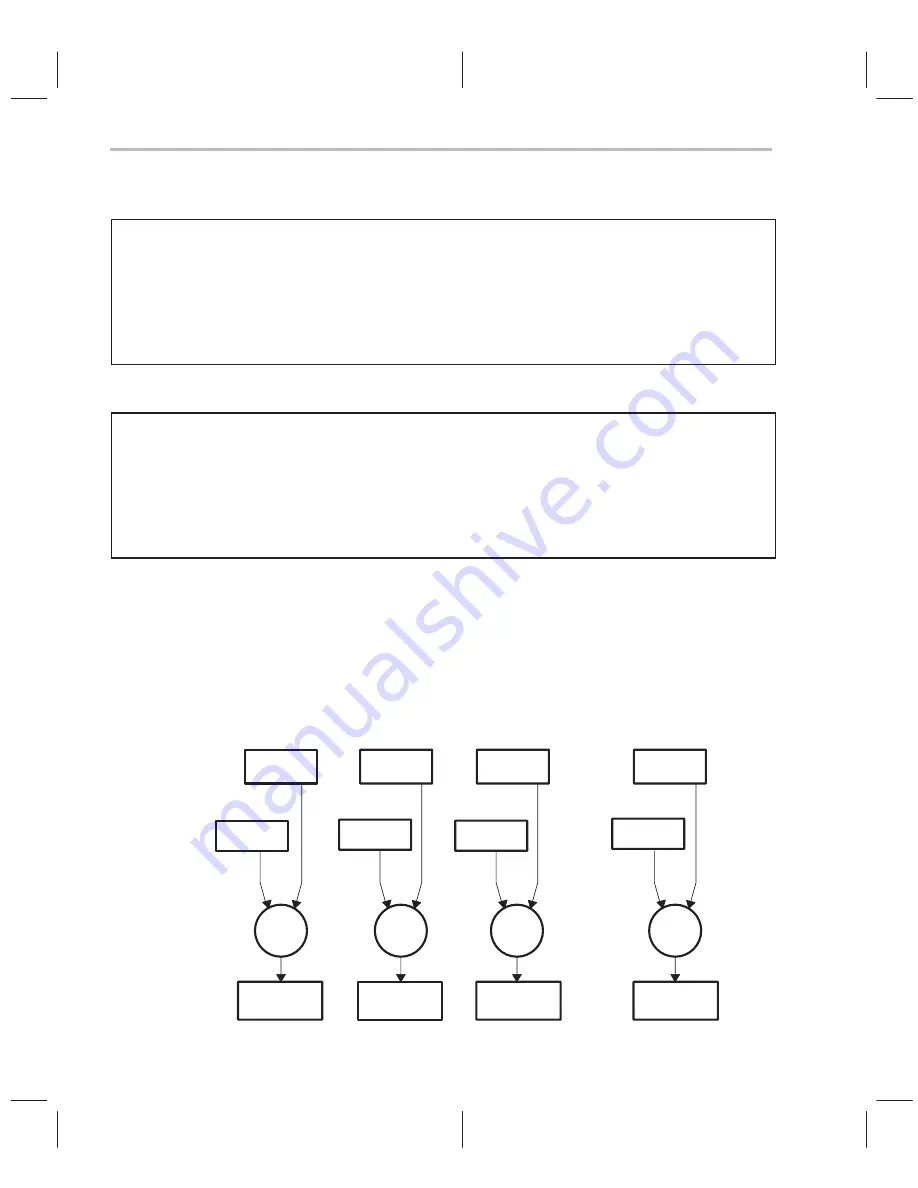
Packed-Data Processing on the ’C64x
8-14
Example 8–1. Vector Sum
void vec_sum(const short *restrict a, const short *restrict b,
short *restrict c, int len)
{
int i;
for (i = 0; i < len; i++)
c[i] = b[i] + a[i];
}
Example 8–2. Vector Multiply
void vec_mpy(const short *restrict a, const short *restrict b,
short *restrict c, int len, int shift)
{
int i;
for (i = 0; i < len; i++)
c[i] = (b[i] * a[i]) >> shift;
}
This type of code is referred to as vector code because each of the input arrays
is a vector of elements, and the same operation is being applied to each ele-
ment. Pure vector code has no computation between adjacent elements when
calculating results. Also, input and output arrays tend to have the same num-
ber of elements. Figure 8–8 illustrates the general form of a simple vector op-
eration that operates on inputs from arrays A and B, producing an output, C
(such as our Vector Sum and Vector Multiply kernels above perform).
Figure 8–8. Graphical Representation of a Simple Vector Operation
Item 0
Item 1
Item n
Item 2
Item 1
Item 0
Item 2
Item n
Item 1
Item 0
Item 2
Item n
(oper)
. . .
. . .
. . .
Input A
Input B
Output C
(oper)
(oper)
(oper)