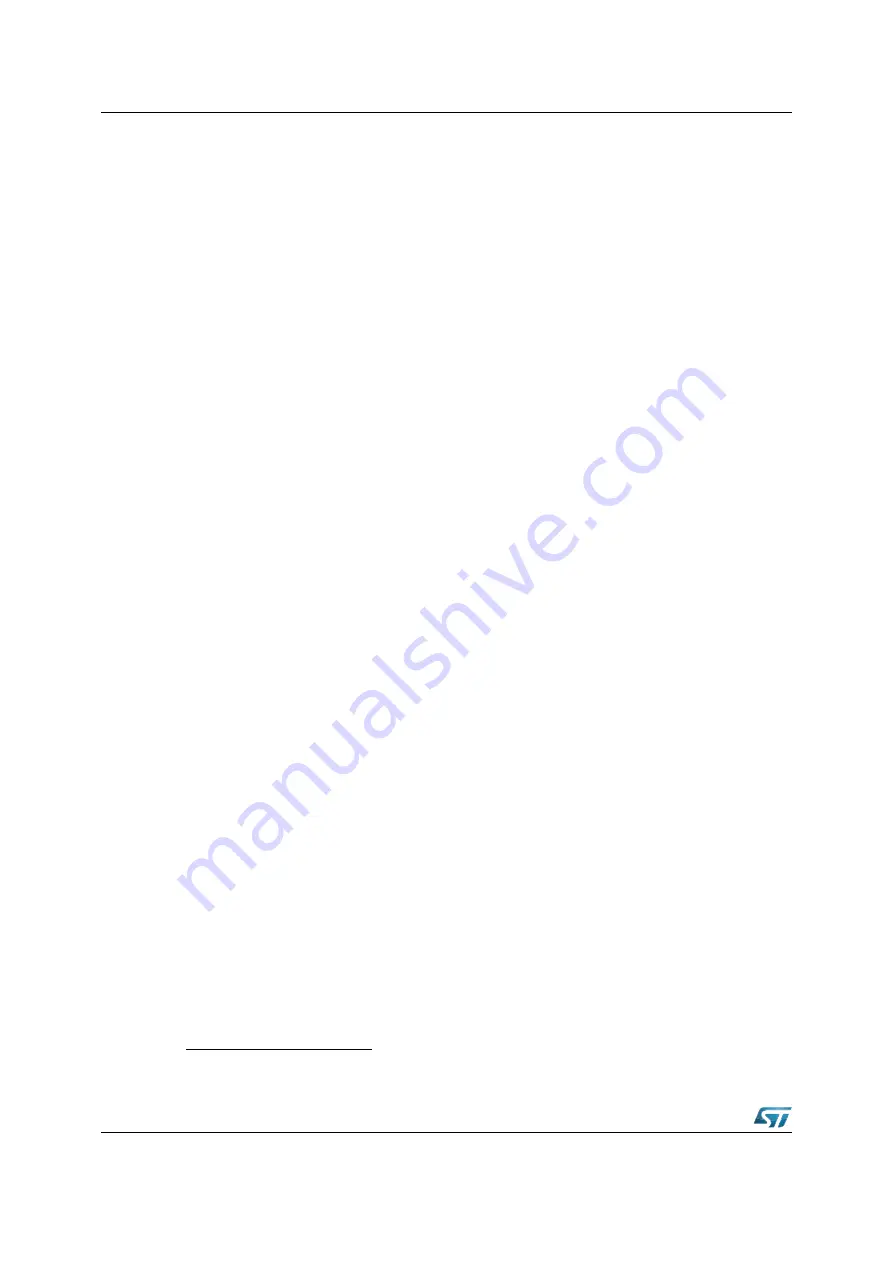
Firmware description
UM1619
36/56
DocID024383 Rev 1
6.6.4 Acknowledgment
Frames
These frames are particular data frames sent to an external device connected to the COMM
module, not allowed to be sent via PLM. They are used by the GUI interface to acknowledge
the commands sent to the module.
From the COMM interface module (SPI, USART, USB, etc.)
uint8_t *buffer;
buffer[0] = 12;
// Error frame payload length (12)
buffer[1] = APP_ACK_FRAME;
// ACK frame type
buffer[2,3] = target_module.group;
// Target device group (2 bytes)
buffer[4,5,6,7] = target_module.address;
// Target device address (4 bytes)
buffer[8] = (
APP_ftype_t
)ack_group_type;
// Frame type to acknowledge
buffer[9] = command_echo;
// Acknowledged command echo
buffer[10,11] = CRC16;
// CRC-16
From / to communication interface (PLM, SPI, USART, USB, etc.)
APP_userdata_t frame;
frame.source = SOURCE_PLM / ...;
// Data source PLM, ...
frame.type = APP_ACK_FRAME;
// ACK frame type
frame.len = 2;
// ACK frame payload length
frame.broadcast = FALSE;
// ACK frames are sent in unicast
frame.group = target_module.group;
// Target device group (2 bytes)
frame.address = target_module.address;
// Target device address (4 bytes)
frame.data[0] = (
APP_ftype_t
)ack_group_type;
// Frame type to acknowledge
frame.data[1] = command_echo;
// Acknowledged command echo
(service, programming, ...)
6.6.5 Programming
Frames
Programming frames are used to program the PLM module parameters, as the static
address, the AESkey (if encryption is used), the data link stack working model and to clear
or read programming parameters. Normally these commands are sent locally to the module
from an external device connected to the COMM peripheral (SPI, USART, USB, etc.).
From the COMM interface module (SPI, USART, USB, etc.)
uint8_t *buffer;
buffer[0] = n + 11;
// Programming frame payload length (n + 11)
buffer[1] = APP_PROGRAMMING_FRAME;
// Programming frame type
buffer[2,3] = target_module.group;
// Target device group (2 bytes)
buffer[4,5,6,7] = target_module.address;
// Target device address (4 bytes)
buffer[8] = (
APP_PROG_CMD_t
)command;
// Programming command
buffer[9,..9+n-1] = programming_data[n];
// Programming data
buffer[9+n,9+n+1] = CRC16;
// CRC-16
From / to communication interface (PLM, SPI, USART, USB, etc.)
APP_userdata_t frame;
frame.source = SOURCE_PLM / ...;
// Data source PLM, ...
frame.type = APP_PROGRAMMING_FRAME;
// Programming frame type
frame.len = n + 1;
// Programming frame payload length
frame.broadcast = TRUE / FALSE;
// TRUE = broadcast, FALSE = unicast
frame.group = target_module.group;
// Programming device group (2 bytes)
frame.address = target_module.address;
// Programming device address (4 bytes)
frame.data[0] = (
APP_PROG_CMD_t
)command;
// Programming command
frame.data[n] = programming_data[n];
// Programming data
Programming commands list
0x01 = PROG_CMD_ENTER_PROG_MODE