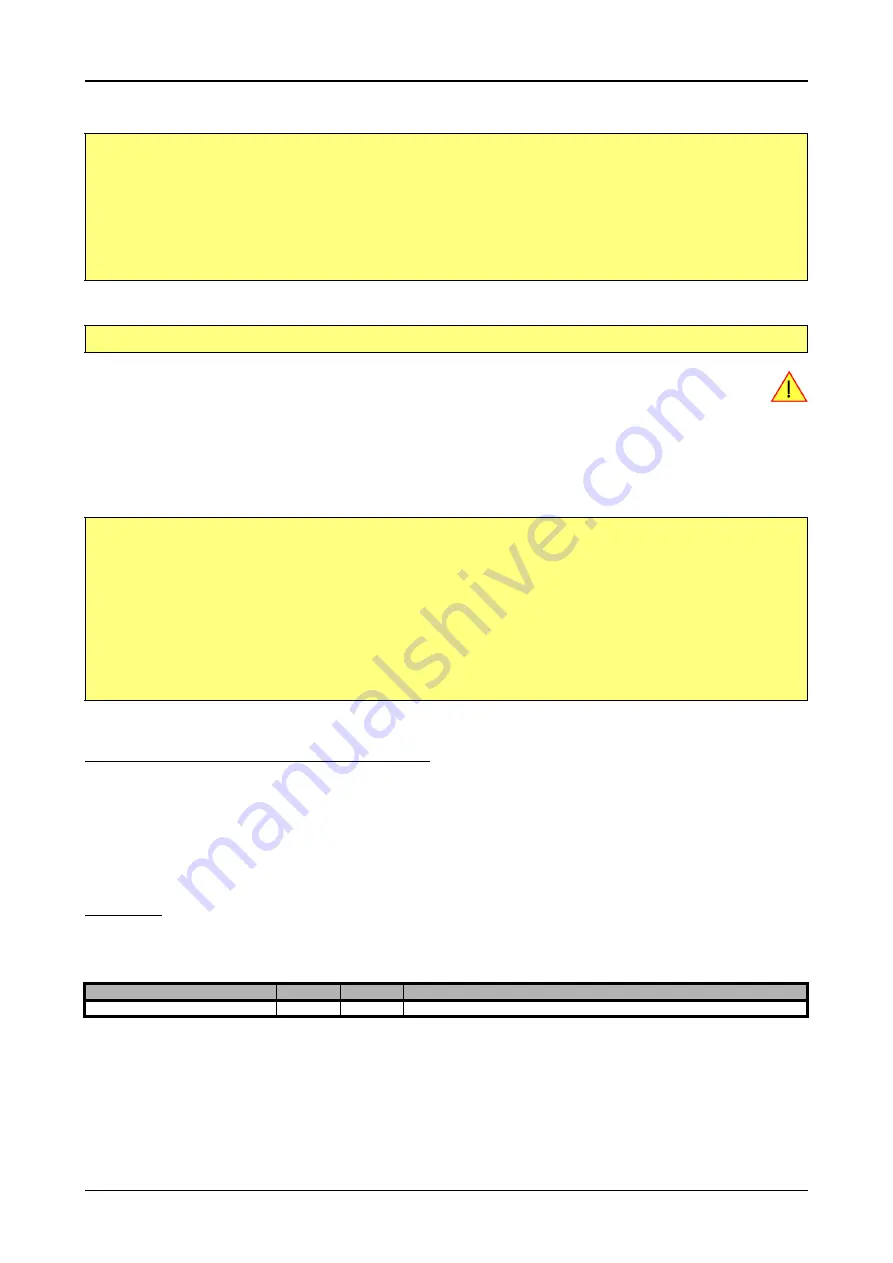
Programming the Board
Gathering information from the card
(c) Spectrum GmbH
57
Example for error checking at end using the error text from the driver:
This short program then would generate a printout as:
All error codes are described in detail in the appendix. Please refer to this error description and the descrip-
tion of the software register to examine the cause for the error message.
Any of the parameters of the spcm_dwGetErrorInfo_i32 function can be used to obtain detailed information on the error. If one is not interested
in parts of this information it is possible to just pass a NULL (zero) to this variable like shown in the example. If one is not interested in the
error text but wants to install its own error handler it may be interesting to just read out the error generating register and value.
Example for error checking with own (simple) error handler:
Gathering information from the card
When opening the card the driver library internally reads out a lot of information from the on-board eeprom. The driver also offers additional
information on hardware details. All of this information can be read out and used for programming and documentation. This chapter will
show all general information that is offered by the driver. There is also some more information on certain parts of the card, like clock machine
or trigger machine, that is described in detail in the documentation of that part of the card.
All information can be read out using one of the spcm_dwGetParam functions. Please stick to the “Driver Functions” chapter for more details
on this function.
Card type
The card type information returns the specific card type that is found under this device. When using multiple cards in one system it is highly
recommended to read out this register first to examine the ordering of cards. Please don’t rely on the card ordering as this is based on the
BIOS, the bus connections and the operating system.
One of the following values is returned, when reading this register. Each card has its own card type constant defined in regs.h. Please note
that when reading the card information as a hex value, the lower word shows the digits of the card name while the upper word is a indication
for the used bus type.
char szErrorText[ERRORTEXTLEN];
spcm_dwSetParam_i64 (hDrv, SPC_SAMPLERATE, 1000000); // correct command
spcm_dwSetParam_i32 (hDrv, SPC_MEMSIZE, -345); // faulty command
spcm_dwSetParam_i32 (hDrv, SPC_POSTTRIGGER, 1024); // correct command
if (spcm_dwGetErrorInfo_i32 (hDrv, NULL, NULL, szErrorText) != ERR_OK) // check for an error
{
printf (szErrorText); // print the error text
spcm_vClose (hDrv); // close the driver
exit (0); // and leave the program
}
Error ocurred at register SPC_MEMSIZE with value -345: value not allowed
uint32 dwErrorReg;
int32 lErrorValue;
uint32 dwErrorCode;
spcm_dwSetParam_i64 (hDrv, SPC_SAMPLERATE, 1000000); // correct command
spcm_dwSetParam_i32 (hDrv, SPC_MEMSIZE, -345); // faulty command
spcm_dwSetParam_i32 (hDrv, SPC_POSTTRIGGER, 1024); // correct command
dwErrorCode = spcm_dwGetErrorInfo_i32 (hDrv, &dwErrorReg, &lErrorValue, NULL);
if (dwErrorCode) // check for an error
{
printf (“Errorcode: %d in register %d at value %d\n”, lErrorCode, dwErrorReg, lErrorValue);
spcm_vClose (hDrv); // close the driver
exit (0); // and leave the program
}
Register
Value
Direction
Description
SPC_PCITYP
2000
read
Type of board as listed in the table below.