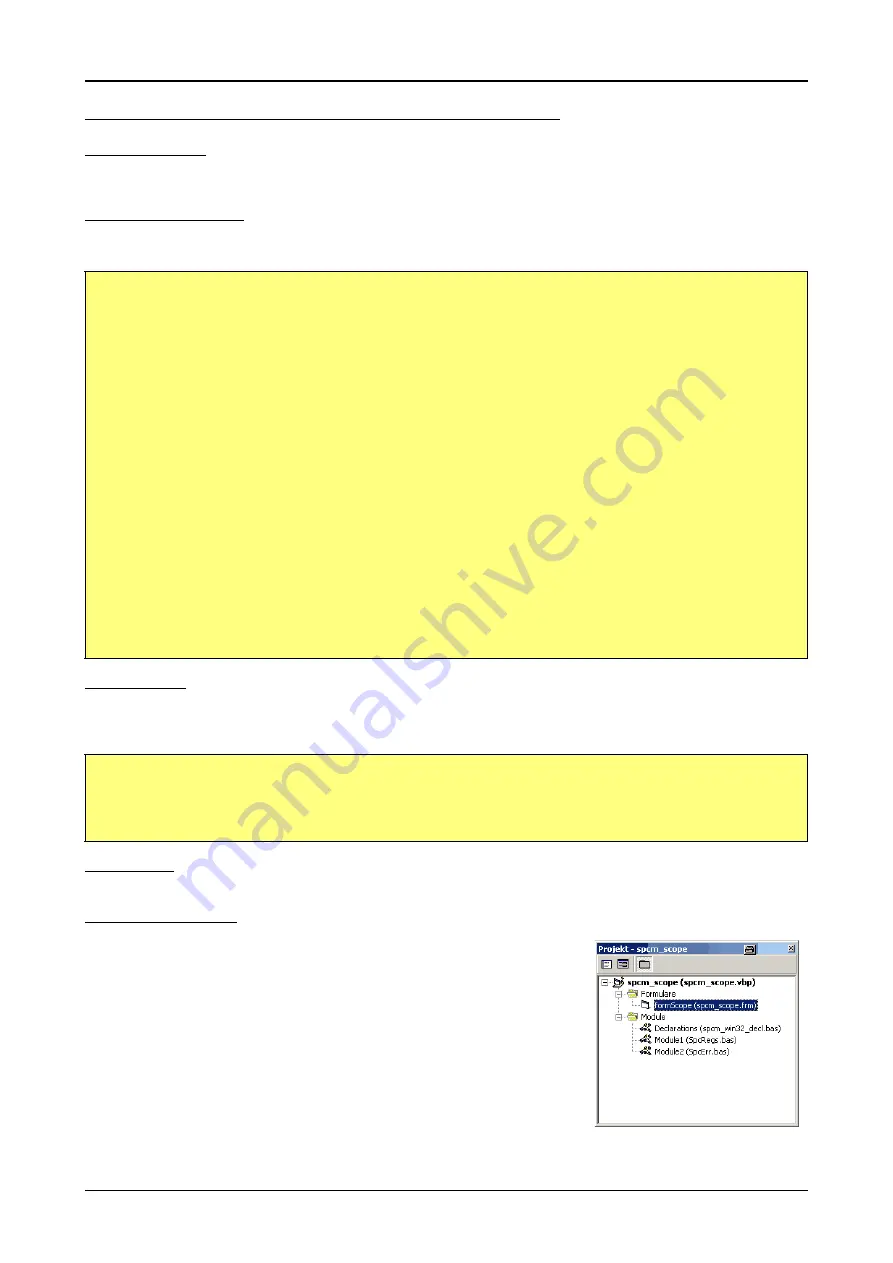
Software
Visual Basic Programming Interface and Examples
(c) Spectrum GmbH
49
Visual Basic Programming Interface and Examples
Driver interface
The driver interface is located in the sub-directory b_header and contains the following files. The files need to be included in the basic project.
Please do not edit any of these files as they’re regularly updated if new functions or registers have been included.
file spcm_win32_decl.bas
The file contains the interface to the driver library and defines some needed constants. All functions of the visual basic library are similar to
the above explained standard driver functions:
file SpcRegs.bas
The SpcRegs.bas file defines all constants that are used for the driver. The constant names are the same names as used under the C/C++
examples. All constants names will be found throughout this hardware manual when certain aspects of the driver usage are explained. It is
recommended to only use these constant names for better visibility of the programs:
file SpcErr.bas
The SpcErr.bas file contains all error codes that may be returned by the driver.
Including the driver files
To use the driver function and all the defined constants it is necessary to include the files into the
project as shown in the picture on the right. The project overview is taken from one of the examples
delivered on CD.
' ----- card handling functions -----
Public Declare Function spcm_hOpen Lib "spcm_win32.dll" Alias "_spcm_hOpen@4"
(ByVal szDeviceName As String) As Long
Public Declare Function spcm_vClose Lib "spcm_win32.dll" Alias "_spcm_vClose@4"
(ByVal hDevice As Long) As Long
Public Declare Function spcm_dwGetErrorInfo_i32 Lib "spcm_win32.dll" Alias "_spcm_dwGetErrorInfo_i32@16"
(ByVal hDevice As Long, ByRef lErrorReg, ByRef lErrorValue, ByVal szErrorText As String) As Long
' ----- software register handling -----
Public Declare Function spcm_dwGetParam_i32 Lib "spcm_win32.dll" Alias "_spcm_dwGetParam_i32@12"
(ByVal hDevice As Long, ByVal lRegister As Long, ByRef lValue As Long) As Long
Public Declare Function spcm_dwGetParam_i64m Lib "spcm_win32.dll" Alias "_spcm_dwGetParam_i64m@16"
(ByVal hDevice As Long, ByVal lRegister As Long, ByRef lValueHigh As Long, ByRef lValueLow As Long) As Long
Public Declare Function spcm_dwSetParam_i32 Lib "spcm_win32.dll" Alias "_spcm_dwSetParam_i32@12"
(ByVal hDevice As Long, ByVal lRegister As Long, ByVal lValue As Long) As Long
Public Declare Function spcm_dwSetParam_i64m Lib "spcm_win32.dll" Alias "_spcm_dwSetParam_i64m@16"
(ByVal hDevice As Long, ByVal lRegister As Long, ByVal lValueHigh As Long, ByVal lValueLow As Long) As Long
' ----- data handling -----
Public Declare Function spcm_dwDefTransfer_i64m Lib "spcm_win32.dll" Alias "_spcm_dwDefTransfer_i64m@36"
(ByVal hDevice As Long, ByVal dwBufType As Long, ByVal dwDirection As Long, ByVal dwNotifySize As Long, ByRef
pvDataBuffer As Any, ByVal dwBrdOffsH As Long, ByVal dwBrdOffsL As Long, ByVal dwTransferLenH As Long, ByVal
dwTransferLenL As Long) As Long
Public Declare Function spcm_dwInvalidateBuf Lib "spcm_win32.dll" Alias "_spcm_dwInvalidateBuf@8"
(ByVal hDevice As Long, ByVal lBuffer As Long) As Long
Public Const SPC_M2CMD = 100 ' write a command
Public Const M2CMD_CARD_RESET = &H1& ' hardware reset
Public Const M2CMD_CARD_WRITESETUP = &H2& ' write setup only
Public Const M2CMD_CARD_START = &H4& ' start of card (including writesetup)
Public Const M2CMD_CARD_ENABLETRIGGER = &H8& ' enable trigger engine
...