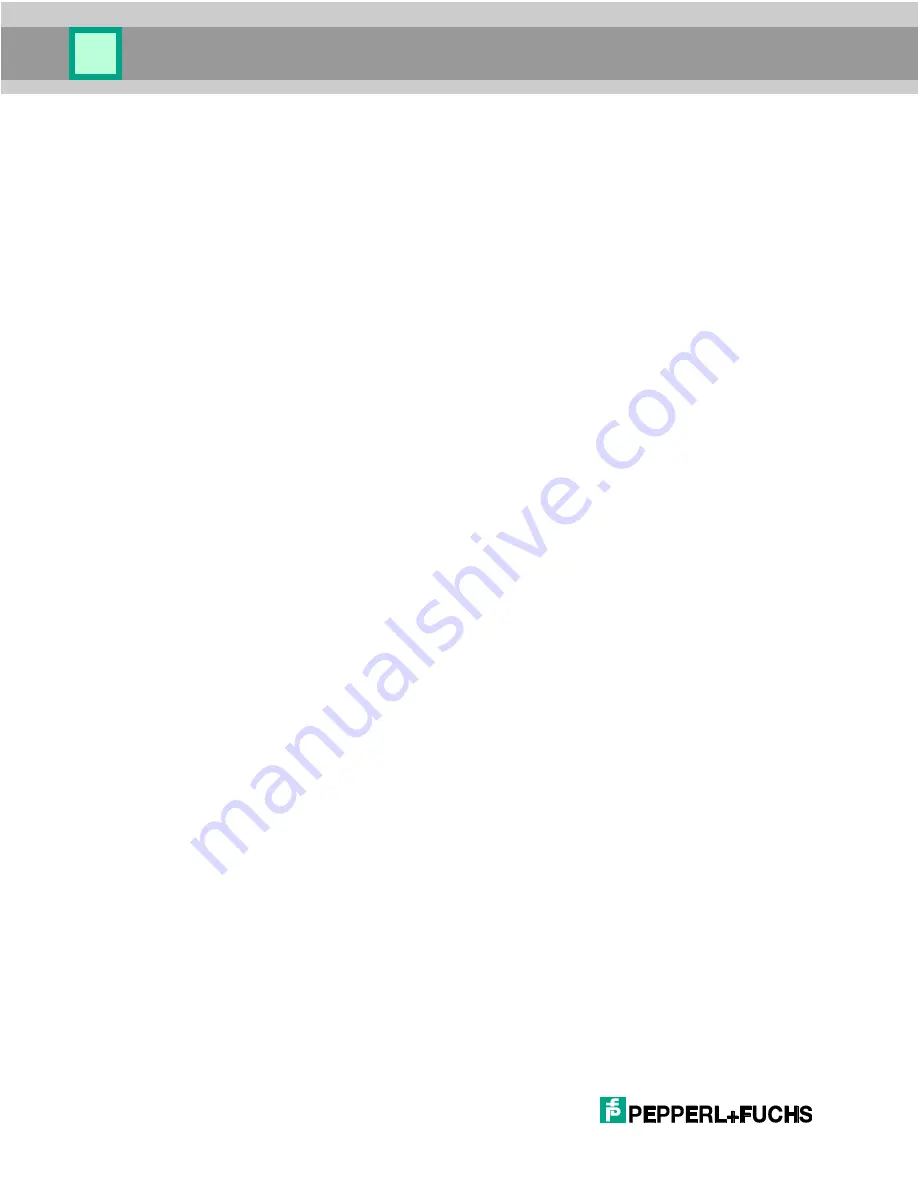
16
Programming Concepts
3.2.1
Softkey Implementation
Softkeys are general purpose, programmable keys. The softkeys are independent of the
GUI display. The gui.showForm, gui.showMenu, and gui.showSubmenu methods include
softkey definitions appropriate for the implementation.
The following example shows the basic approach to programming the softkeys and
implementing their event handlers.
// define send-key functions used by common softkeys
function sendEnter() { gui.sendKey(gui.key.enter); }
function sendEscape() { gui.sendKey(gui.key.escape); }
// create some common softkeys
var selectSoftkey = new gui.Softkey("Select", sendEnter);
var okSoftkey = new gui.Softkey("OK", sendEnter);
var backSoftkey = new gui.Softkey("Back", sendEscape);
var cancelSoftkey = new gui.Softkey("Cancel", sendEscape);
See section 0 (gui object) for more information.
3.2.2
Forms
Forms are the building blocks of your application. Each form represents a set of actions
you want to present to the user on screen.
Use the gui.Form object (section 4.2.3.3) to define the forms for your application. Section
4.2.3 defines the form object and several constructors that you can use to create controls
on your application form.
The following examples demonstrate how to create a form. The event handler functions
need to be defined for your application.
// JavaScript Form Demo Script Document
// form event handlers
function myFormOnOk(){/* processing code (example: save the
Employee #) */}
function myFormOnCancel(){/* processing code (example: return to
main menu) */}
// create the form object
var myForm = new gui.Form(myFormOnOk, myFormOnCancel);
// create the edit control
var edit = new gui.Edit("");
// create the label control
var label = new gui.Label("Employee #:");
// position the controls on the form