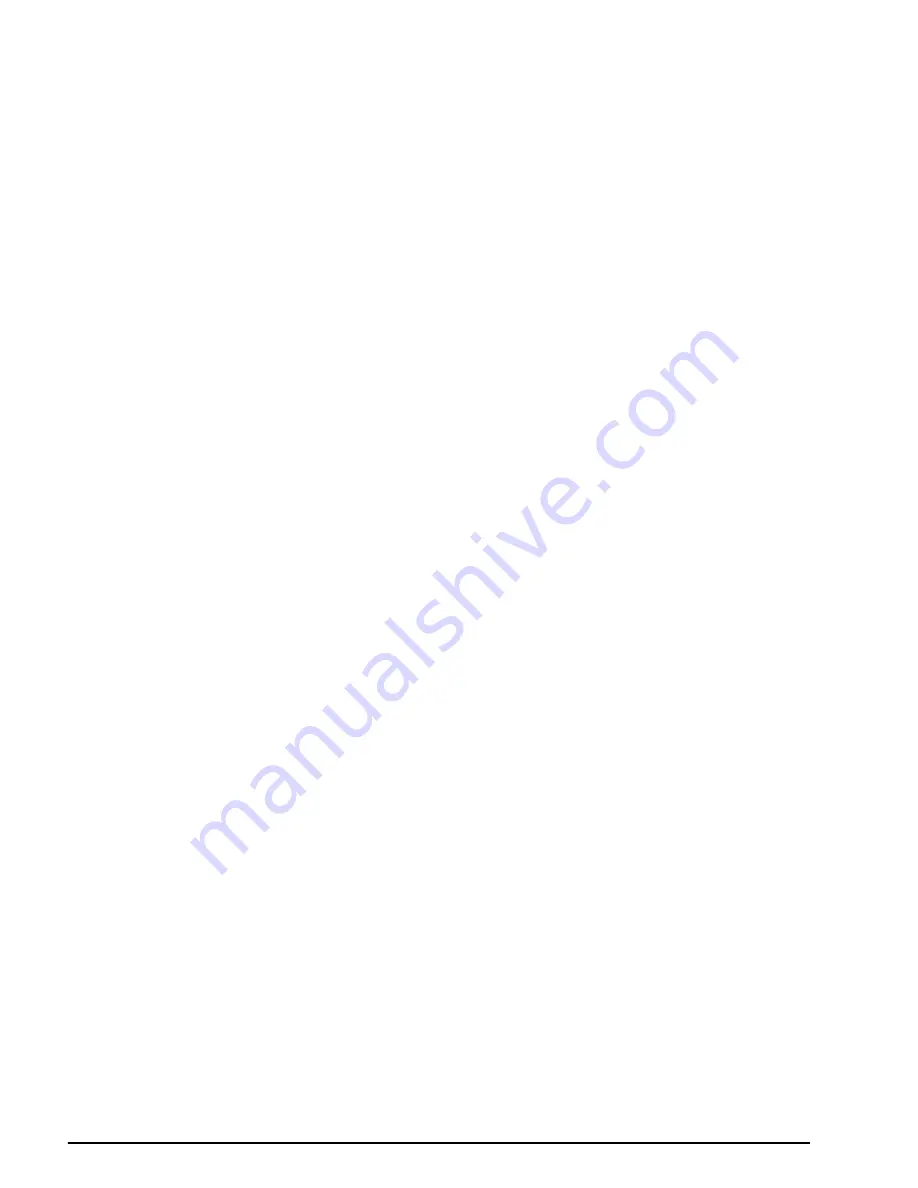
6
| MODBUS & CAMPBELL SCIENTIFIC DATALOGGERS | GEOKON
'Main Program
BeginProg
'Open COM port with RS-485 communications at 115200 baud rate
SerialOpen (ComC1,115200,16,0,50,3)
'Read all 5 sensors in string every 4 seconds
Scan (4,Sec,0,0)
'Loop to read each thermistor
For Count = 1 To 5
'Reset temporary storage for next reading
Res = 0
'Flush Serial between readings
SerialFlush (ComC1)
'Write to register 0x118 to trigger thermistor string
'NOTE: ModbusMaster won't send 0x118 unless "&H119" is entered
ModbusMaster (ErrorCode,ComC1,115200,Count,6,1,&H119,1,1,50,0)
'Delay after triggering the measurement
Delay (1,1,Sec)
'Use Modbus command to retrieve resistance from thermistor string
ModbusMaster (ErrorCode,ComC1,115200,Count,3,Res,&H103,1,1,50,0)
'Calculate thermistor temperature from ohms to Celsius using Steinhart-hart equation
Celsius(Count) = 1/(A+B* LN(Res) + C * LN(Res)^3)-273.15
Next
'Call Table to store Data
CallTable Test
NextScan
EndProg
4.4
SAMPLE CR1000 PROGRAM WITH REQUIRED MODEL 8020-38
ADDRESSABLE BUS CONVERTER
The following sample program reads one 3810A string with five addressable
thermistor sensors. The string in this example is connected to a RS-485 to TTL
converter and communicates with the CR1000 through the control ports C1 and
C2, which are setup as COM1:
'Constants used in Steinhart-Hart equation to calculate sensor temperature for
'10k thermistor
'Define Data Tables
'Main Program
Const A = 1.128706256E
-3
Const B = 2.342327483E
-4
Const C = 0.8707279757E
-7
Public ErrorCode
'Result of ModBusMaster communications attempt
Public Resistance As Float
'Resistance of thermistor must be stored as Type Float
Public Celsius(5)
'Calculated Celsius for 5 sensors in string
Public Count
'Counter to increment through temperature sensors
DataTable(Test,1,-1)
Sample (5,Celsius(),IEEE4)
'Sample Celsius for 5 sensors in string
EndTable
BeginProg
'Open COM port with TTL communications at 115200 baud rate
SerialOpen (Com1,115200,16,0,50)
'Read all 5 sensors in string every 4 seconds
Scan (4,Sec,0,0)
'Loop to read each thermistor
For Count = 1 To 5
'Reset temporary storage for next reading
Resistance = 0
'Flush Serial between readings
SerialFlush (Com1)
'Write to register 0x118 to trigger thermistor string
'NOTE: ModbusMaster won't send 0x118 unless "&H119" is entered
ModbusMaster (ErrorCode,Com1,115200,Count,6,1,&H119,1,1,50,0)
'Delay after triggering the measurement
Delay (1,1,Sec)
'Use Modbus command to retrieve resistance from thermistor string
ModbusMaster (ErrorCode,Com1,115200,Count,3,Resistance,&H103,1,1,50,0)
'Calculate thermistor temperature from ohms to Celsius using Steinhart-hart equation
Celsius(Count) = 1/(A+B* LN(Resistance) + C * LN(Resistance)^3)-273.15
Next
'Call Table to store data
CallTable Test
NextScan
EndProg