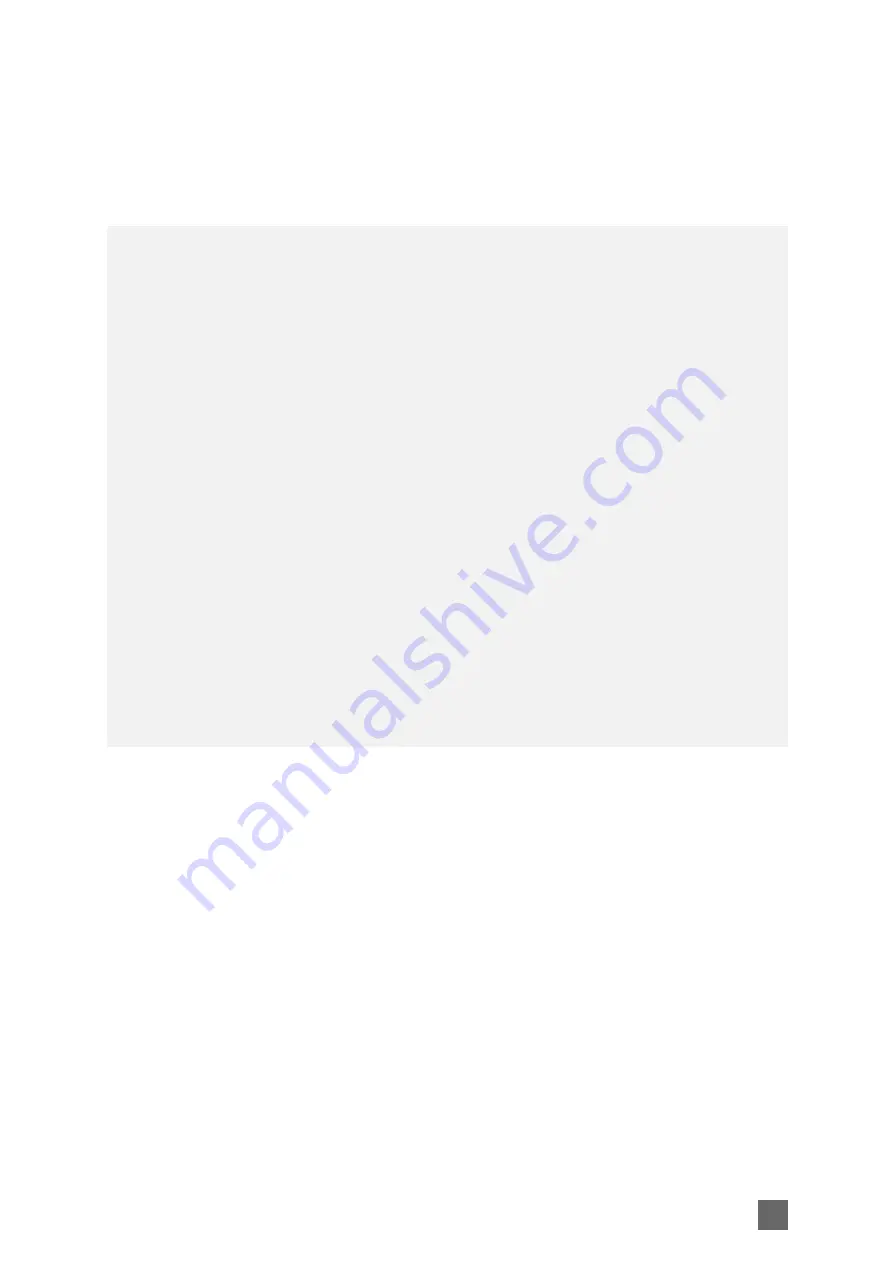
29
New buffer callbacks
This program shows how to access information related to
new buffer
events. It uses
CallbackMultiThread
, but it could use another callback method just as well.
#include <iostream>
#include <EGrabber.h>
using namespace Euresys;
class MyGrabber : public EGrabber<CallbackMultiThread> {
public:
MyGrabber(EGenTL &gentl) : EGrabber<CallbackMultiThread>(gentl) {
runScript("config.js");
enableEvent<NewBufferData>();
reallocBuffers(3);
start();
}
private:
virtual void onNewBufferEvent(const NewBufferData &data) {
ScopedBuffer buf(*this, data);
// 1
uint64_t ts = buf.getInfo<uint64_t>(GenTL::BUFFER_INFO_TIMESTAMP);
// 2
std::cout << "event timestamp: " << data.timestamp << " us, "
// 3
<< "buffer timestamp: " << ts << " us" << std::endl;
}
// 4
};
int main() {
try {
EGenTL gentl;
MyGrabber grabber(gentl);
while (true) {
}
} catch (const std::exception &e) {
std::cout << "error: " << e.what() << std::endl;
}
}
1. In
onNewBufferEvent
, create a temporary
ScopedBuffer
object
buf
. The
ScopedBuffer
constructor takes two arguments:
n
the grabber owning the buffer: since we are in a class derived from
EGrabber
, we simply
pass
*this
;
n
information about the buffer: this is provided in
data
.
2. Retrieve the timestamp of the buffer, which is defined as the time at which
the camera
started to send data to the frame grabber
.
3. As explained in the section about
,
new buffer
events are slightly different
from the other kinds of events: they are standard (as per GenTL), and don't have an
associated
numid
.
As a consequence, the
NewBufferData
structure passed to
onNewBufferEvent
doesn't
have a
numid
field. It does, however, have a
timestamp
field indicating the time at which
the driver was notified that data transfer to the buffer was complete
. This
event timestamp
is
inevitably greater than the
buffer timestamp
retrieved in step 2.
5. Euresys::EGrabber
Coaxlink
Programmer Guide