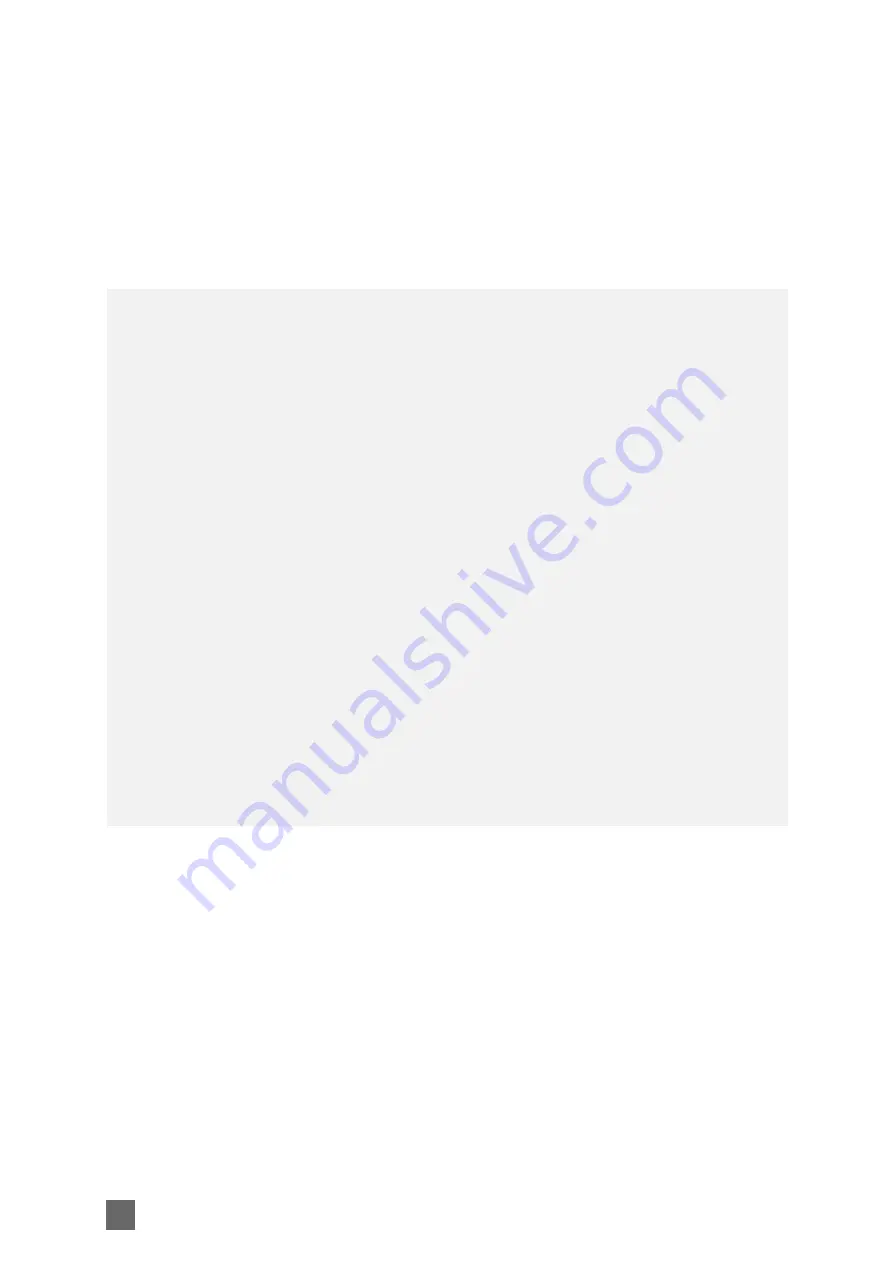
26
5.6. Events and callbacks examples
On demand callbacks
This program displays basic information about CIC events generated by a grabber:
#include <iostream>
#include <EGrabber.h>
using namespace Euresys;
// 1
class MyGrabber : public EGrabber<CallbackOnDemand> {
// 2
public:
MyGrabber(EGenTL &gentl) : EGrabber<CallbackOnDemand>(gentl) {
// 3
runScript("config.js");
// 4
enableEvent<CicData>();
// 5
reallocBuffers(3);
start();
}
private:
virtual void onCicEvent(const CicData &data) {
std::cout << "timestamp: " << std::dec << data.timestamp << " us, " // 6
<< "numid: 0x" << std::hex << data.numid
// 6
<< " (" << getEventDescription(data.numid) << ")"
<< std::endl;
}
};
int main() {
try {
EGenTL gentl;
MyGrabber grabber(gentl);
while (true) {
grabber.processEvent<CicData>(1000);
// 7
}
} catch (const std::exception &e) {
std::cout << "error: " << e.what() << std::endl;
}
}
1. This
using
directive allows writing
Xyz
instead of
Euresys::Xyz
. This helps keep lines
relatively short.
2. Define a new class
MyGrabber
which is derived from
EGrabber<CallbackOnDemand>
.
3.
MyGrabber
's constructor initializes its base class by calling
EGrabber<CallbackOnDemand>
's constructor.
4. Run a
config.js
script which should:
n
properly configure the camera and frame grabber;
n
enable
for CIC events.
5. Enable
onCicEvent
callbacks.
Coaxlink
Programmer Guide
5. Euresys::EGrabber