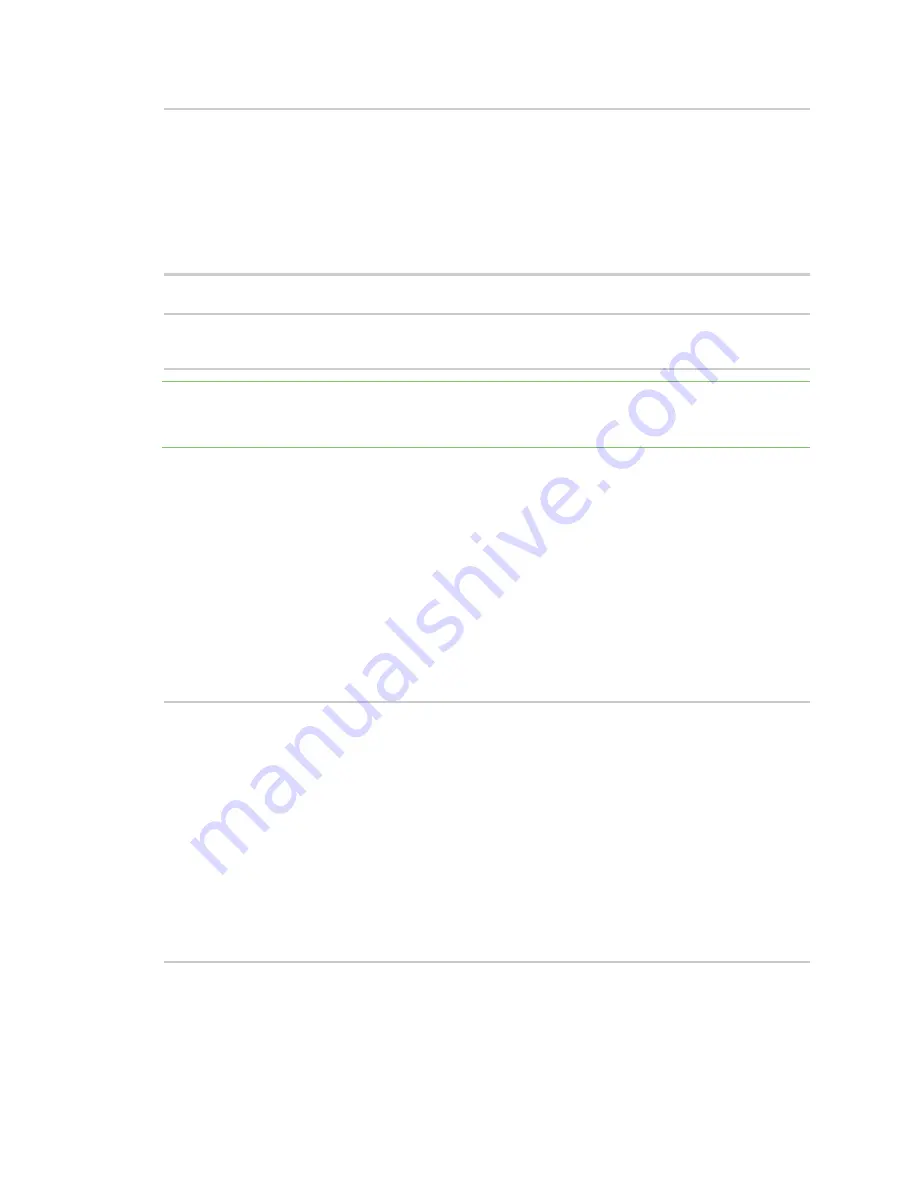
Get started with MicroPython
MicroPython examples
Digi XBee® 3 802.15.4 RF Module User Guide
45
import xbee, time
while True:
print("Receiving data...")
print("Press CTRL+C to cancel.")
p = xbee.receive()
if p:
format_packet(p)
else:
time.sleep(0.25)
# wait 0.25 seconds before checking again
If this node had previously received a packet, it outputs as follows:
Unicast message from EUI-64 00:13:a2:00:41:74:ca:70 (network 0x6D81)
from EP 0xE8 to EP 0xE8, Cluster 0x0011, Profile 0xC105:
b'Hello World!'
Note
Digi recommends calling the
receive()
function in a loop so no data is lost. On modules where
there is a high volume of network traffic, there could be data lost if the messages are not pulled from
the queue fast enough.
Example: communication between two XBee 3 802.15.4 modules
This example combines all of the previous examples and represents a full application that configures a
network, discovers remote nodes, and sends and receives messages.
First, we will upload some utility functions into the flash space of MicroPython so that the following
examples will be easier to read.
Complete the following steps to compile and execute utility functions using flash mode on both
devices:
1. Access the MicroPython environment.
2. Press
Ctrl + F
.
3. Copy the following code:
import xbee, time
# Utility functions to perform XBee 3 802.15.4 operations
def format_eui64(addr):
return ':'.join('%02x' % b for b in addr)
def format_packet(p):
type = 'Broadcast' if p['broadcast'] else 'Unicast'
print("%s message from EUI-64 %s (network 0x%04X)" %
(type, format_eui64(p['sender_eui64']), p['sender_nwk']))
print("from EP 0x%02X to EP 0x%02X, Cluster 0x%04X, Profile 0x%04X:" %
(p['source_ep'], p['dest_ep'], p['cluster'], p['profile']))
print(p['payload'],"\n")
def network_status():
# If the value of AI is non zero, the module is not connected to a network
return xbee.atcmd("AI")
4. At the MicroPython 1^^^ prompt, right-click and select the
Paste
option.
5. Press
Ctrl+D
to finish. The code is uploaded to the flash memory and then compiled. At the
"Automatically run this code at startup" [Y/N]?" prompt, select
Y
.