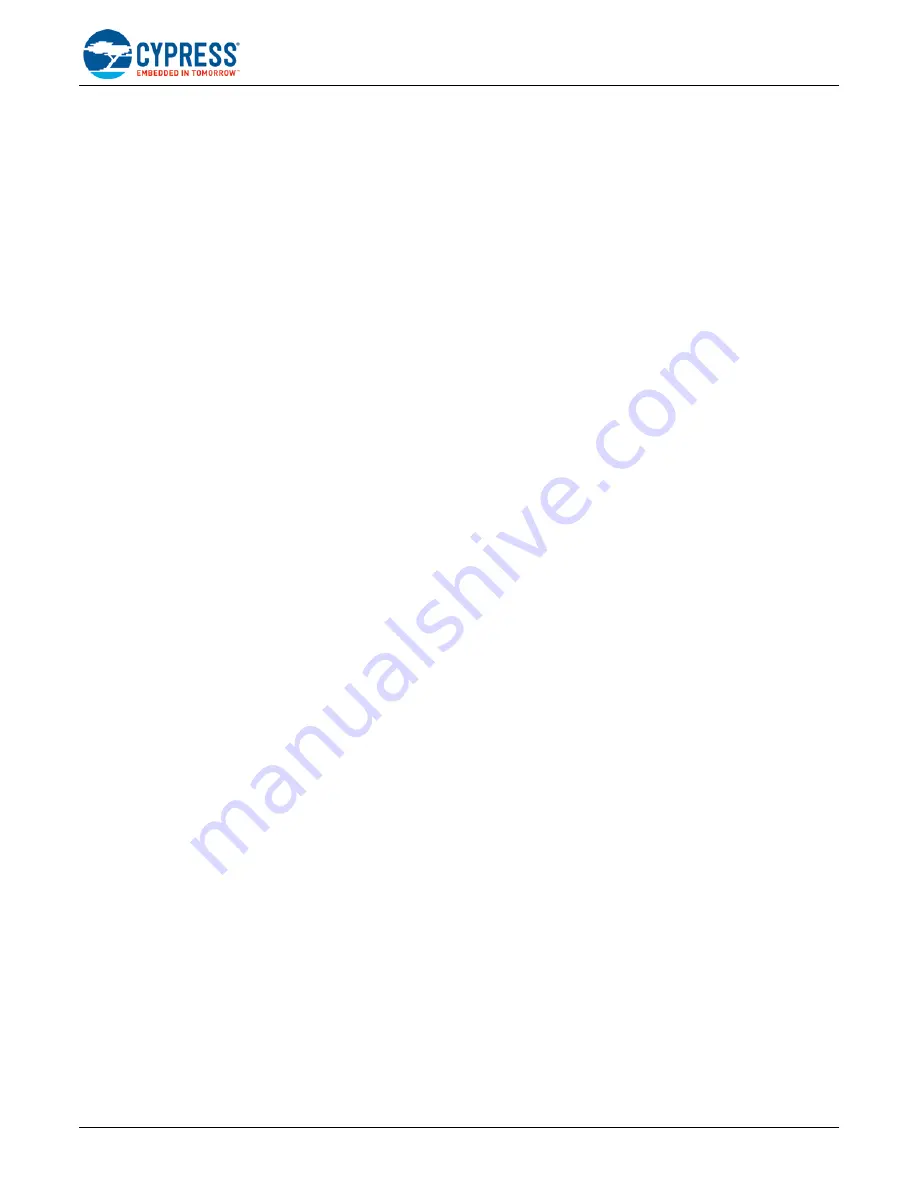
Interface Programming Information and Examples
CYW920706WCDEVAL Hardware User Guide Doc. No.: 002-16535 Rev. **
41
10.5 PUART Programming Example
The following example shows how to initialize the peripheral UART. The complete working project for this example can be
found in WICED Studio under Project Explorer
20706-A2_Bluetooth\apps\snip\hal_puart
. Pertinent sections of source file
hal_puart_app.c
are shown here:
#include "wiced_hal_puart.h"
void puar_rx_interrupt_callback(void* unused)
{
// There can be at most 16 bytes in the HW FIFO.
uint8_t readbyte;
wiced_hal_puart_read( &readbyte );
/* send one byte via the TX line. */
wiced_hal_puart_write( r1 );
if( readbyte == 'S' )
{
/* send a string of characters via the TX line */
wiced_hal_puart_print( "\nYou typed 'S'.\n" );
}
wiced_hal_puart_reset_puart_interrupt( );
}
/* Sample code to test puart driver. Initializes puart, selects puart pads,
* turn off flow control, and enables Tx and Rx.
* Echoes the input byte with increment by 1.
*/
void test_puart_driver( void )
{
uint8_t read_5_bytes[5];
wiced_hal_puart_init( );
// Possible uart tx and rx combination.
// Pin for Rx: p2, Pin for Tx: p0
// Note that p2 and p0 might not be available for use on your
// specific hardware platform.
// Please see the User Documentation to reference the valid pins.
wiced_hal_puart_select_uart_pads( WICED_PUART_RXD, WICED_PUART_TXD, 0, 0);
/* Turn off flow control */
wiced_hal_puart_flow_off( ); // call wiced_hal_puart_flow_on(); to turn on flow
// control
// BEGIN - puart interrupt
wiced_hal_puart_register_interrupt(puar_rx_interrupt_callback);
/* Turn on Tx */
wiced_hal_puart_enable_tx( ); // call wiced_hal_puart_disable_tx to disable
// transmit capability.
wiced_hal_puart_print( "Hello World!\r\nType something! Keystrokes are echoed to
the terminal ...\r\n");
/* Enable to change puart baud rate. eg: 9600, 19200, 38200 */
//wiced_hal_puart_set_baudrate( 115200 );
}