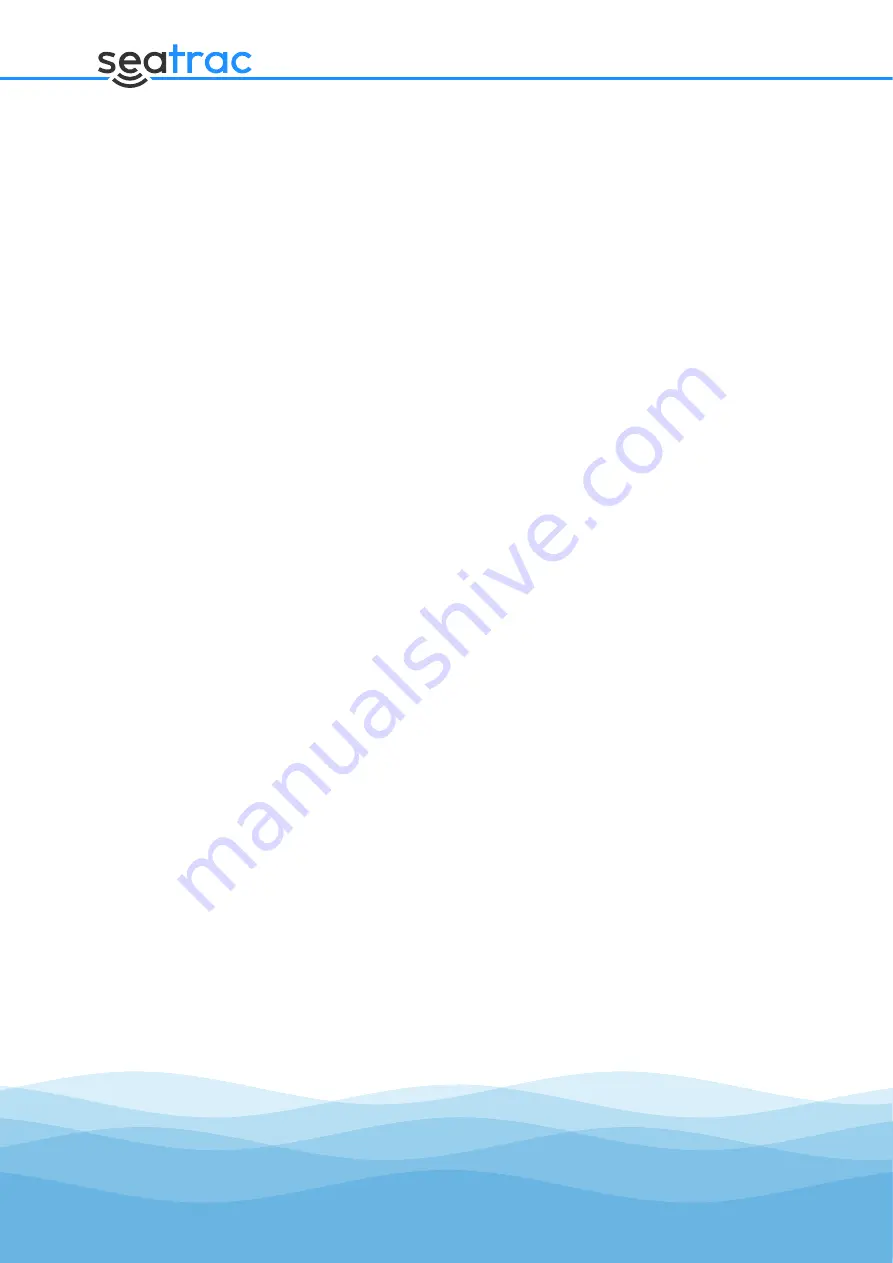
SeaTrac Serial Command Interface Reference
Page 27
5.6.
Checksums
The last 4 characters of each message (prior to the <CR><LF> characters) represent the 16-
bit checksum value (send in Little-Endian form).
Checksums are computed by processing in a sequential byte-wise fashion the messages binary
content after (but not including) the synchronisation character, and not including the
checksum value itself – i.e. the CID and Payload message fields.
This applied both to received and transmitted messages.
Message checksums are generated using the CRC-16-IBM polynomial of
.
(Normal polynomial 0x8005, Reversed polynomial of 0xA001)
1
2
15
16
x
x
x
When receiving a message, the computed checksum should be compared against the received
checksum, and if the two values match then the message is considered valid.
When transmitting a message, a buffer should be populated with the CID and message
payload, then the checksum of this computed and appended to the end of the buffer.
The following C code shows a simple algorithm for computing the CRC of the message buffer…
/*!
Function that computes the CRC16 value for an array of sequential bytes
stored in memory.
NB: Types uint8 and uint16 represent unsigned 8 and 16 bit integers
Respectively.
@param buf Pointer to the start of the buffer to compute the CRC16 for.
@param len The number of bytes in the buffer to compute the CRC16 for.
@result The new CRC16 value.
*/
uint16 CalcCRC16(uint8* buf, uint16 len)
{
uint16 poly = 0xA001;
uint16 crc = 0;
for(uint16 b = 0; b < len; b++) {
uint8 v = *buf;
for(uint8 i = 0; i < 8; i++) {
if((v & 0x01) ^ (crc & 0x01)) {
crc
>>=
1;
crc
^=
poly;
}
else {
crc
>>=
1;
}
v >>= 1;
}
buf++;
}
return crc;
}
The following message strings show typical synchronisation, CID and checksum data, and can
be used to validate checksum algorithm implementations…